Learn the basics of FastAPI, a Python framework for building web APIs. In this course you will build a full-stack fin-tech web application using Plaid and FastAPI.
FastAPI - Crash Course
This walkthrough is meant to be supplemental to the video course on how to use FastAPI.
It mimics the structure of the official FastAPI Tutorial - User Guide documentation where each section is meant to build on the previous one, while also being relevant as a stand-alone topic.
It will briefly cover each topic and then implement each section into a full stack fintech application with Plaid.
If you'd like to go further in depth on a topic then be sure to reference the FastAPI Documentation.
What is FastAPI?
FastAPI is an unopinionated, light-weight framework for building web API's in Python 3.6+. Created by @tiangolo.
Out of the box it offers a ton of features with no extra work.
Asynchronous Support
FastAPI boasts that it is a modern, fast (high-performance), web framework. This is in part due to its support for asynchronous functionality.
Older versions of Python only supported Synchronous behavior.
This meant that, if code within the program had to wait for something else to finish, like fetching data via a network request, it would block the entire program until that request finished, then move onto the next task.
In recent years Python has developed Asynchronous (commonly referred to as async) support.
The beauty of async is that, if you have code that requires waiting for any amount of time, you can tell your program "Hey program! This is going to take a bit, so you should go do other stuff, and then come back when it's done."
This allows the program to perform other things that would normally have to wait until the first task is done.
Python now has built in support for this in the form of the standard library asyncio.
This standardized support in Python allowed the standardization of asynchronous support among web servers as well in the form of the ASGI spec, the successor to WSGI (These are just standards not implementations).
Older web frameworks like Django and Flask were built around WSGI and require additional modifications to enable async support or mimic something like it.
FastAPI is built around Starlette and Uvicorn which were designed to have async support built in.
This is done as easily as adding the async / await
syntax into your code.
@app.get("/items")
async def():
items = await db.get_items()
return {"items": items}
Performance
Independent TechEmpower benchmarks show FastAPI applications running under Uvicorn as one of the fastest Python frameworks available, only below Starlette and Uvicorn themselves (used internally by FastAPI).
-- https://fastapi.tiangolo.com/#performance
See One of the fastest Python frameworks available for more information and benchmarks.
Reduction of code
There is little to no boiler plate to get started. The smallest FastAPI app is just a few lines of code.
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def root():
return { "message": "Smallest API Ever"}
It is designed to be clean, simple and easy to learn.
Based on Standards
Since FastAPI is based on standards like Python's type hints and OpenAPI it plays well with others and is very configurable.
This allows you to only have to declare the types of parameters once and as simply as defining function parameters. You don't have to learn any new syntax. If you know Python you know FastAPI!
class Item:
name: str
attributes: Dict[str, str]
@app.post("/item")
def create_item(item: Item):
return {
"message": f"Sucessfully created new item {item.name}!",
"attributes": item.attributes,
}
This will automatically provide
- Autocompletion and type checks in your editor
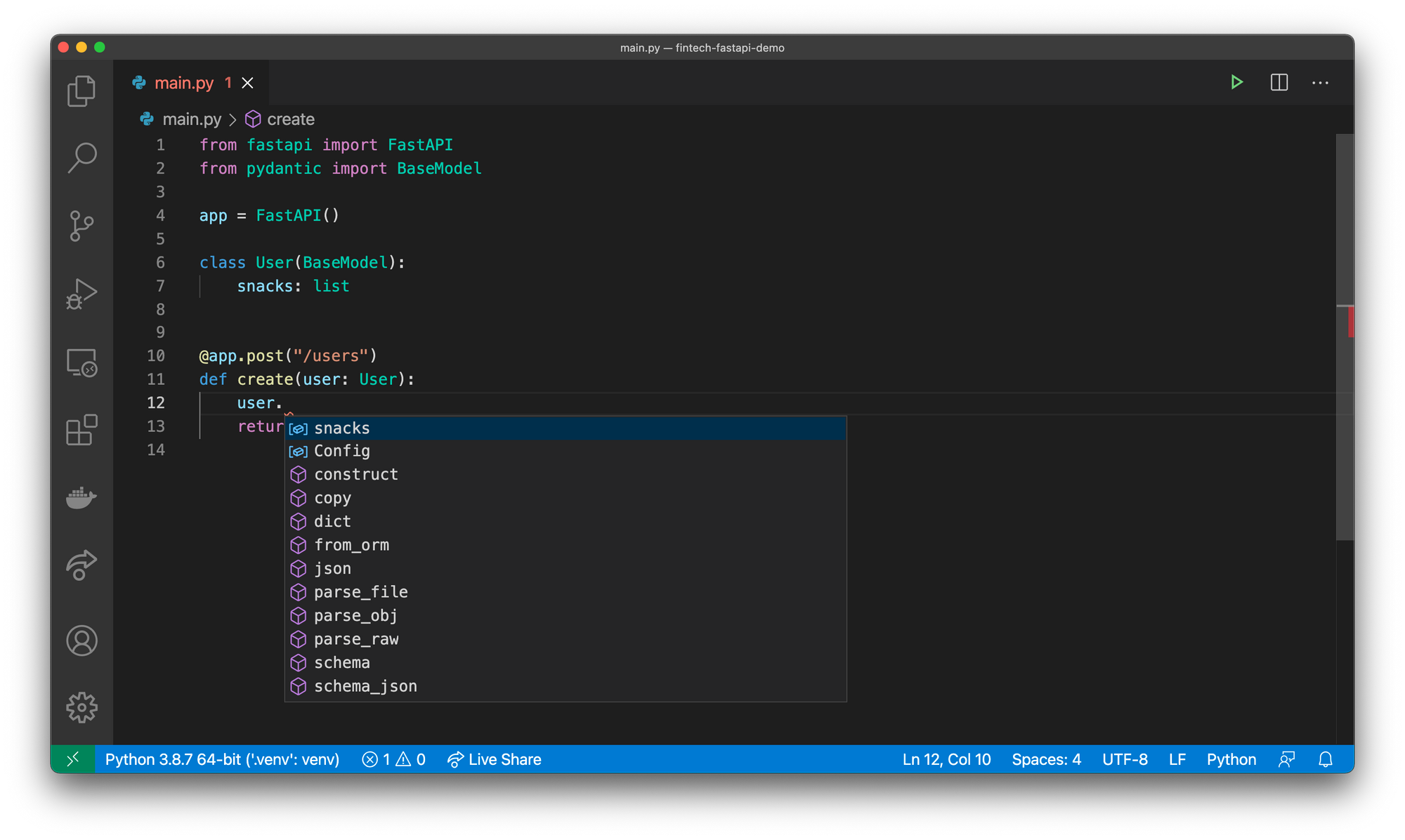
- Validation of incoming and outgoing data.
- Serialization of incoming and outgoing data.
and my personal favorite automatically generated interactive documentation.
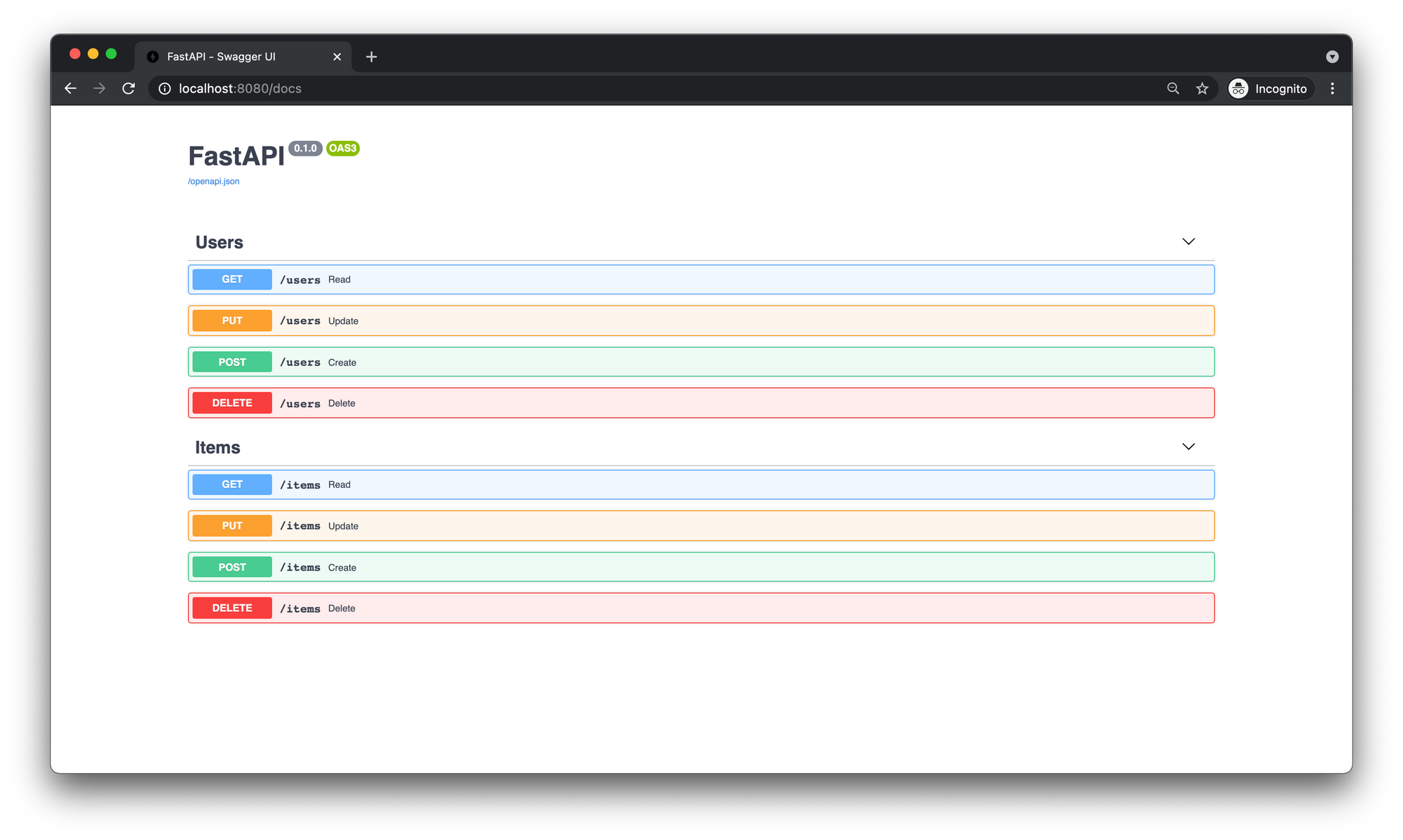
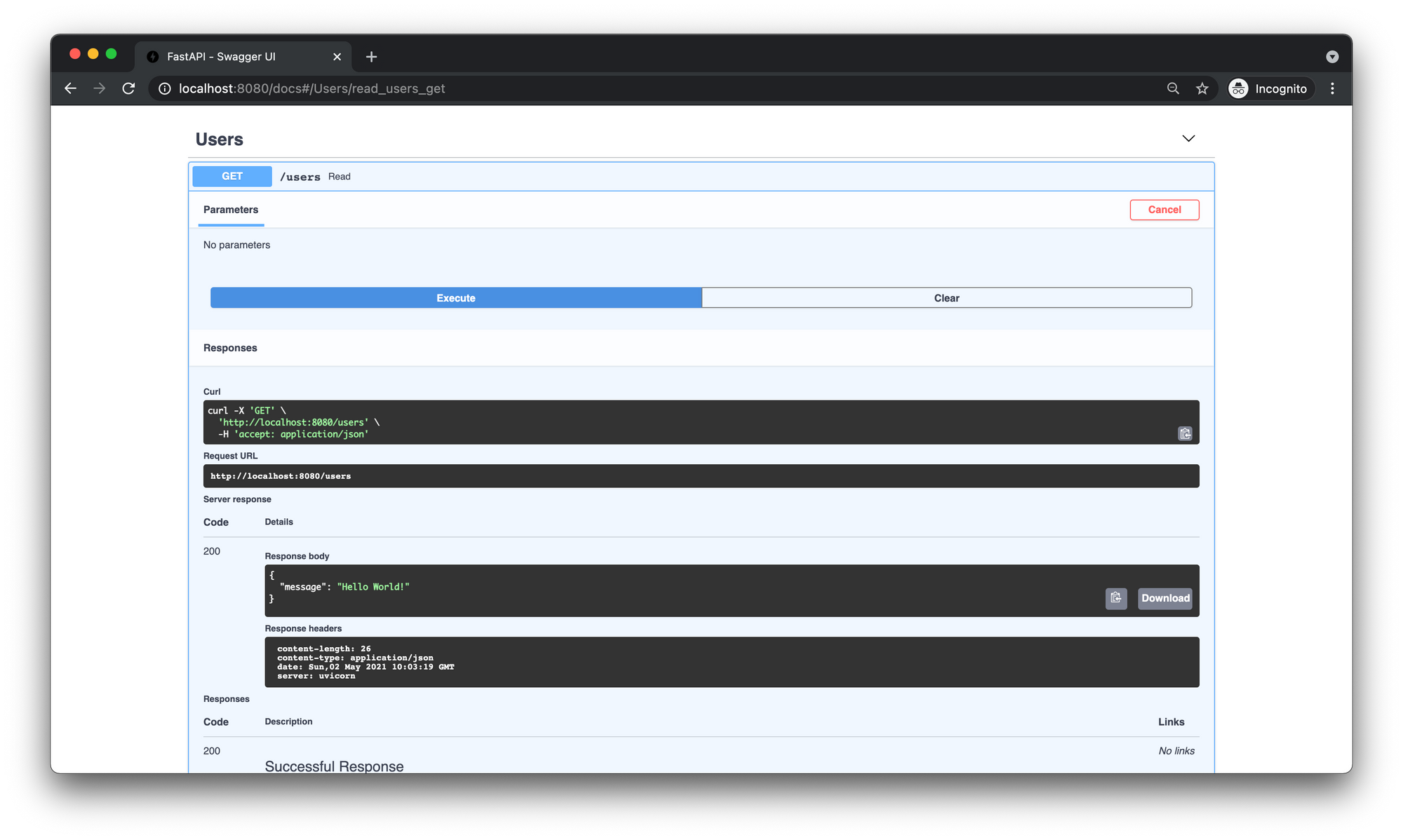
...this is just to name a few! Be sure to read up on all of FastAPI's amazing features.
If you're convinced on FastAPI's awesomeness and want to dive in and get your hands dirty then keep reading!
Build a FastAPI Fintech App with Plaid
So, if you've made it this far and you want to learn about FastAPI while building a really cool fintech project than stick around!
The focus of this course is on FastAPI and we will not be going into depth on any Plaid or Javascript related topics.Plaid
If you don't know what Plaid is chances are, you're using it everyday with at least one of your banking apps. It's used by Venmo, Coinbase, Acorns, American Express and thats just to name a few!
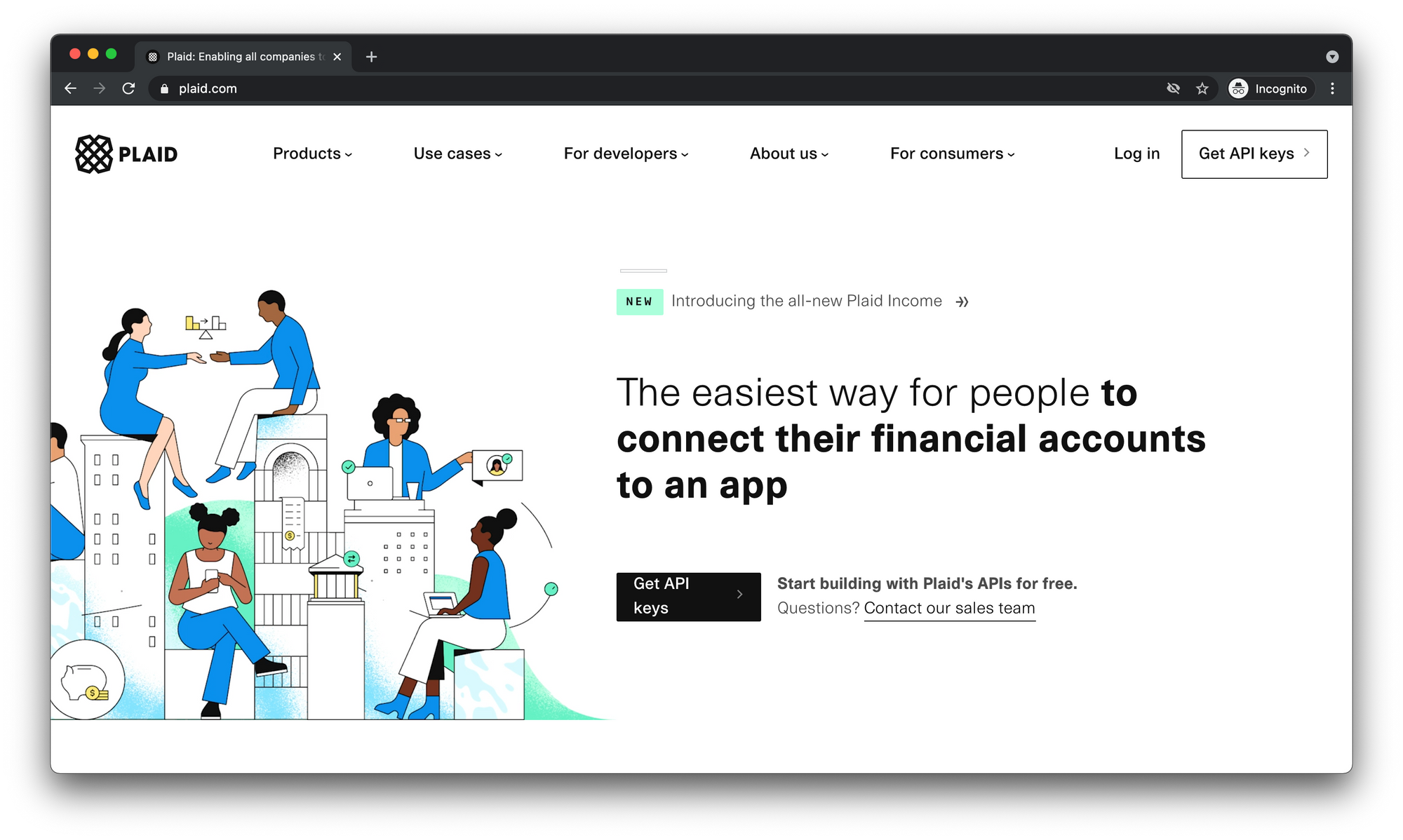
When it comes to banking and fintech, security is obviously the number one concern. Handling the security and authentication between financial institutions is no easy task.
Plaid is a plug-and-play solution to this. It makes it easy for developers to outsource this and allow you to move onto the business logic of building your application.
What We'll Build
The good people at Plaid have created an amazing Quickstart React/Typescript application along with a great tutorial on how to get setup with Plaid in just about every language.
We are going to be using the React/Typescript frontend application and building out our own FastAPI backend for it.
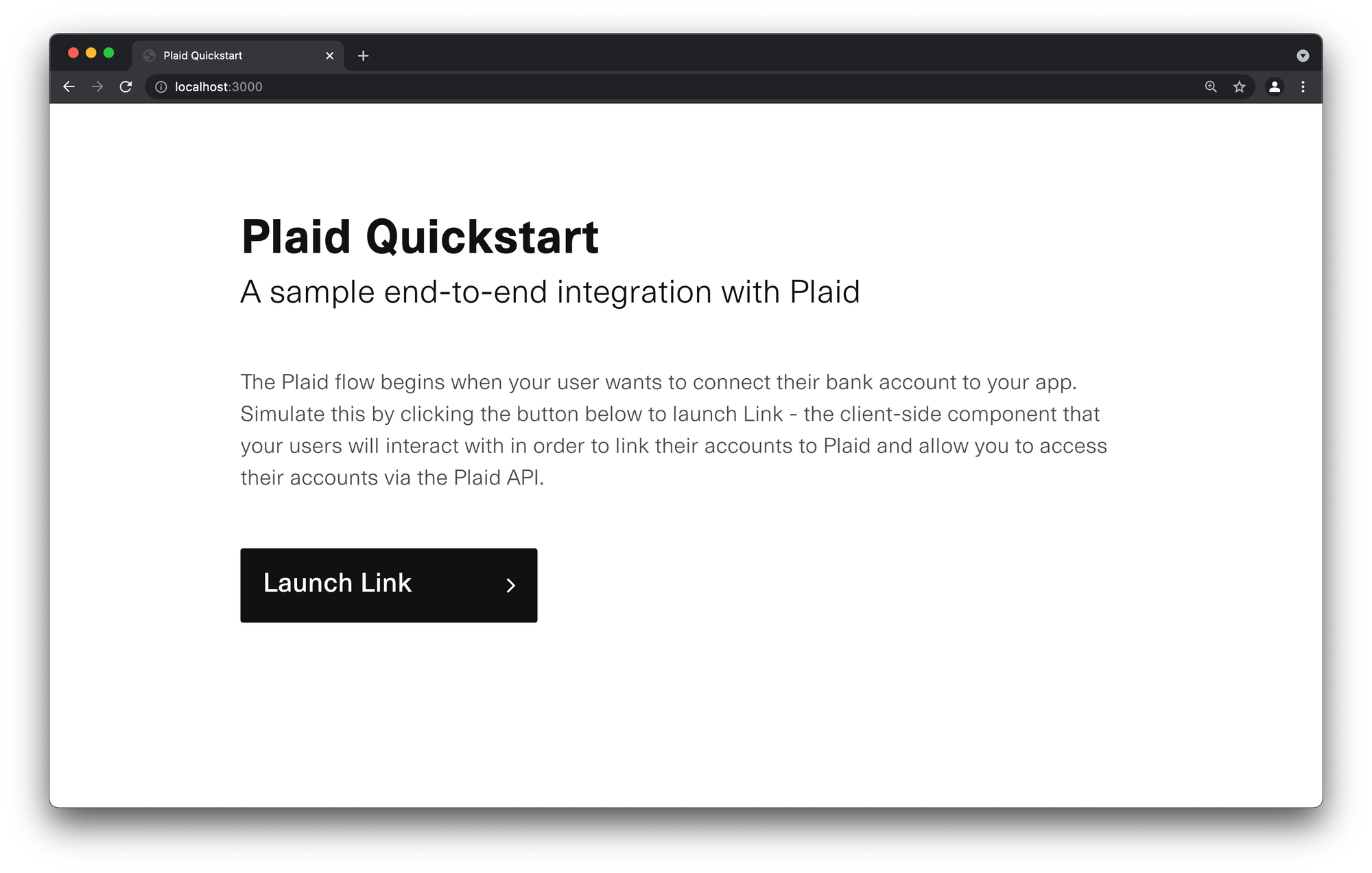
We will implement...
- initial setup and Authentication
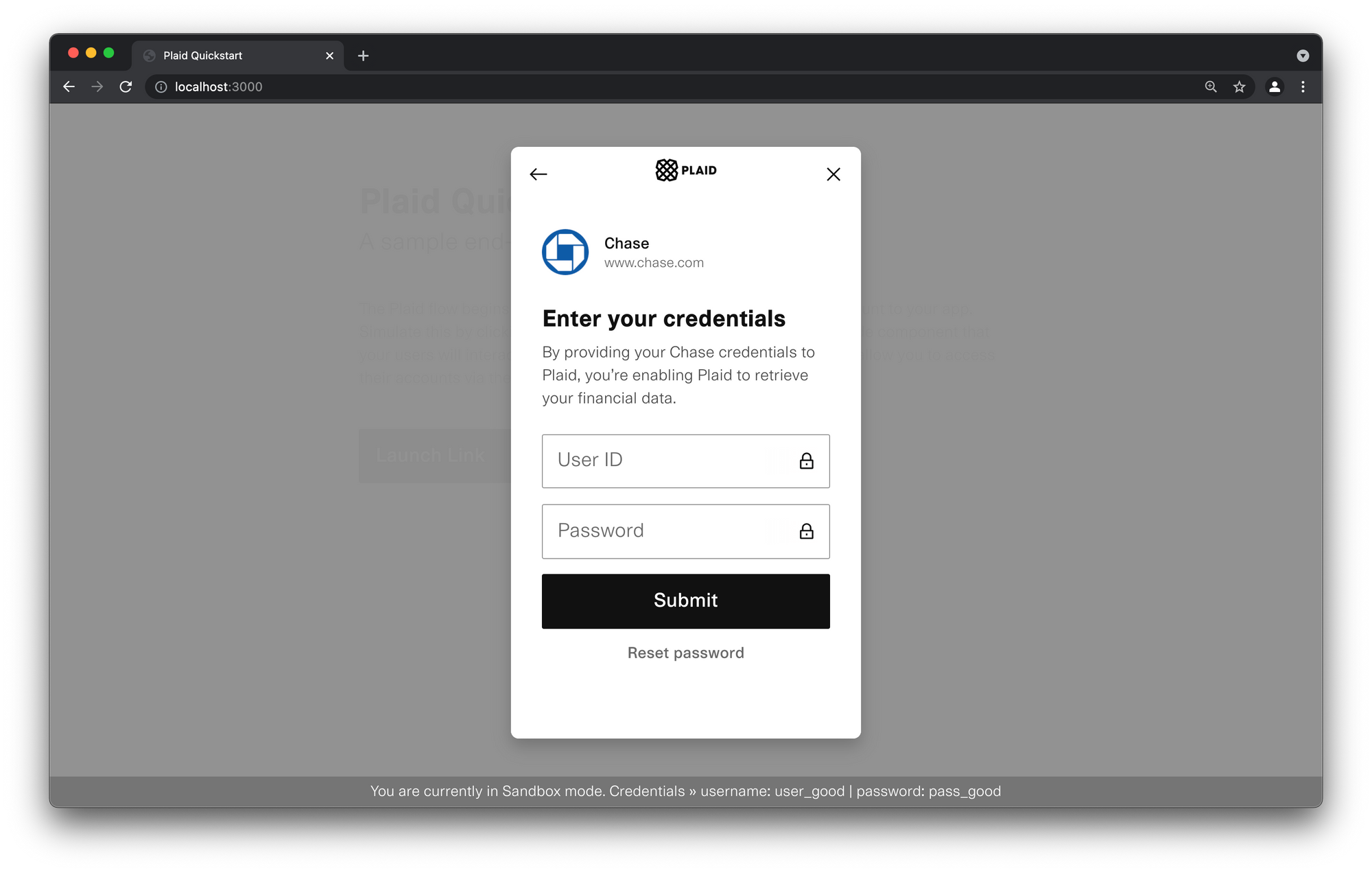
- Creating new Items and Accounts
- Checking Balances
- Fetching Investment Portfolios
- Queries for fetching Transactions
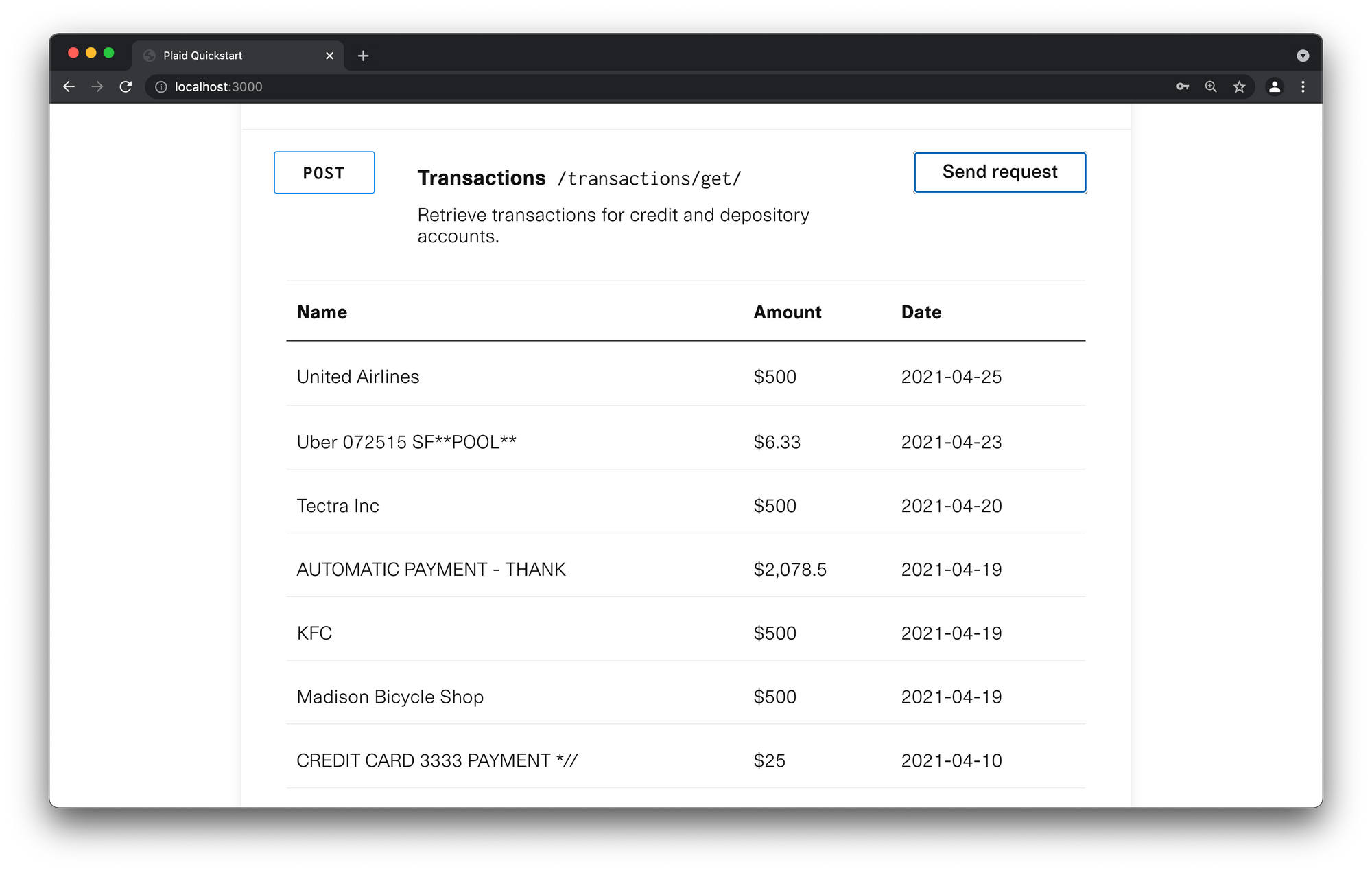
Just to name a few.
Topics we'll cover in FastAPI
A few of the things we'll cover in FastAPI will be...
- Path, and query parameters with validation
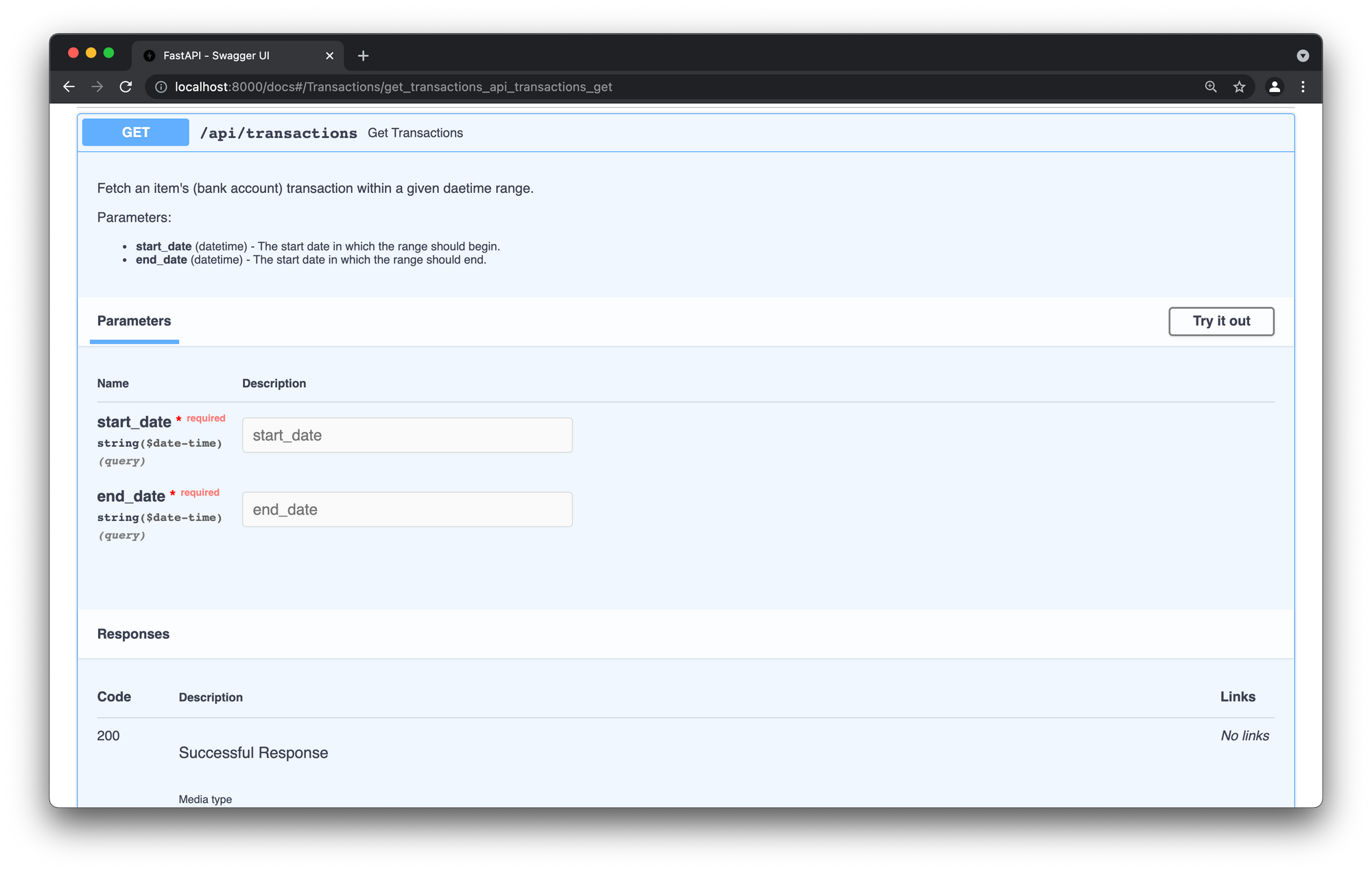
- Creating models for request body validation
- Cookie and header parameters
- Creating response models
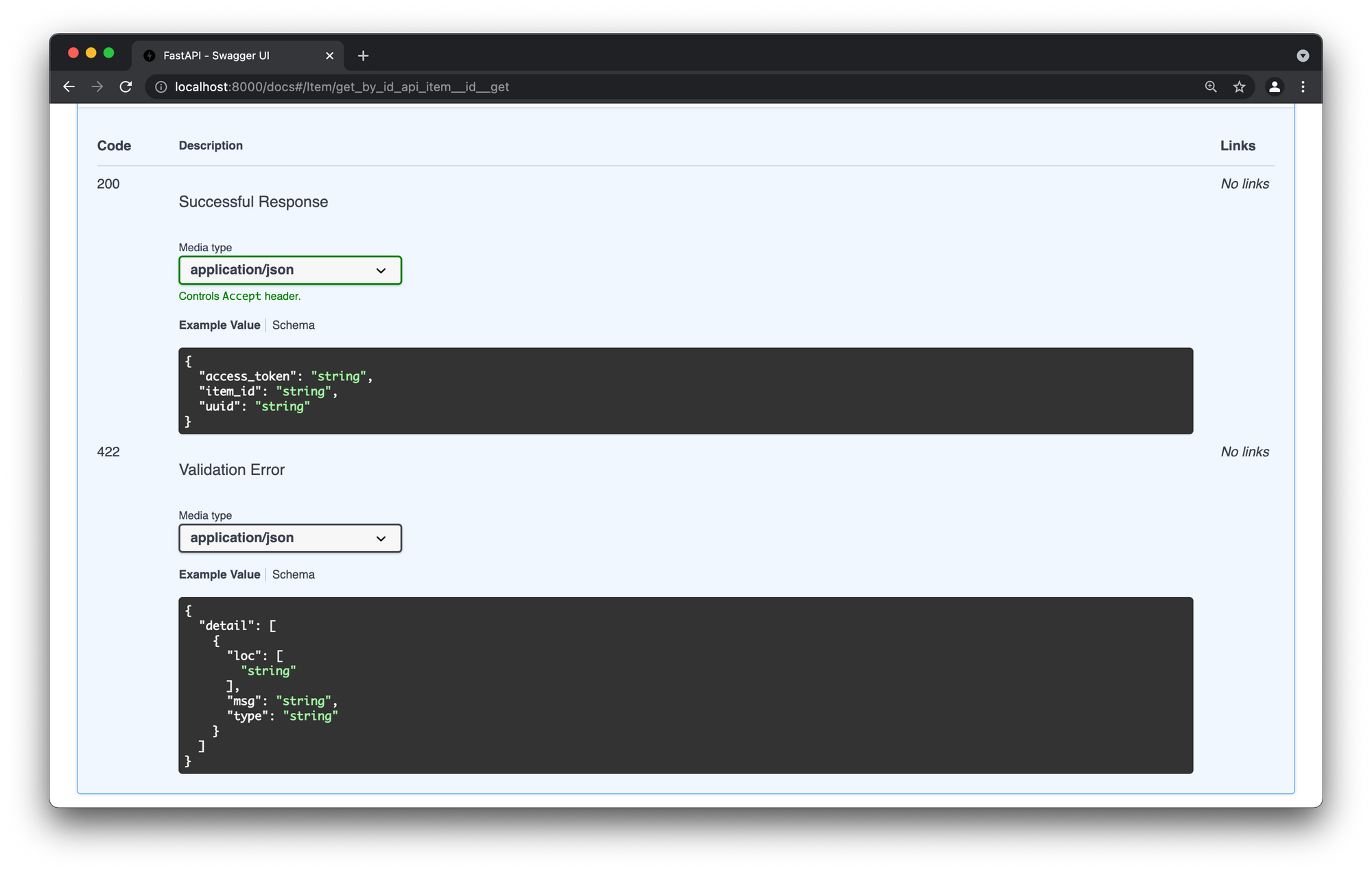
- Error handling
- Middleware and CORS
- Securing our application with OAuth2
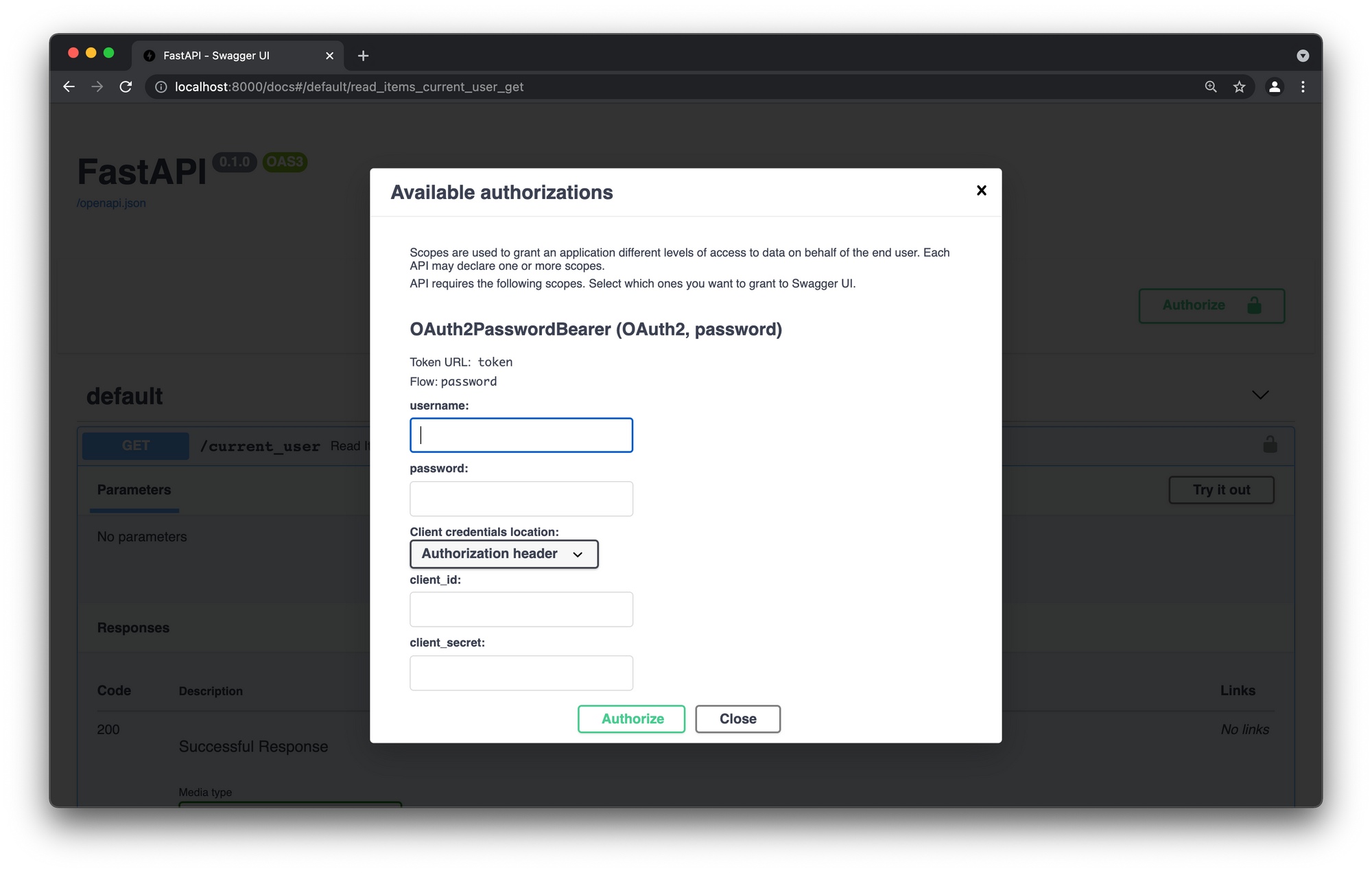
- Handling environment variables
- FastAPI's dependency injection system
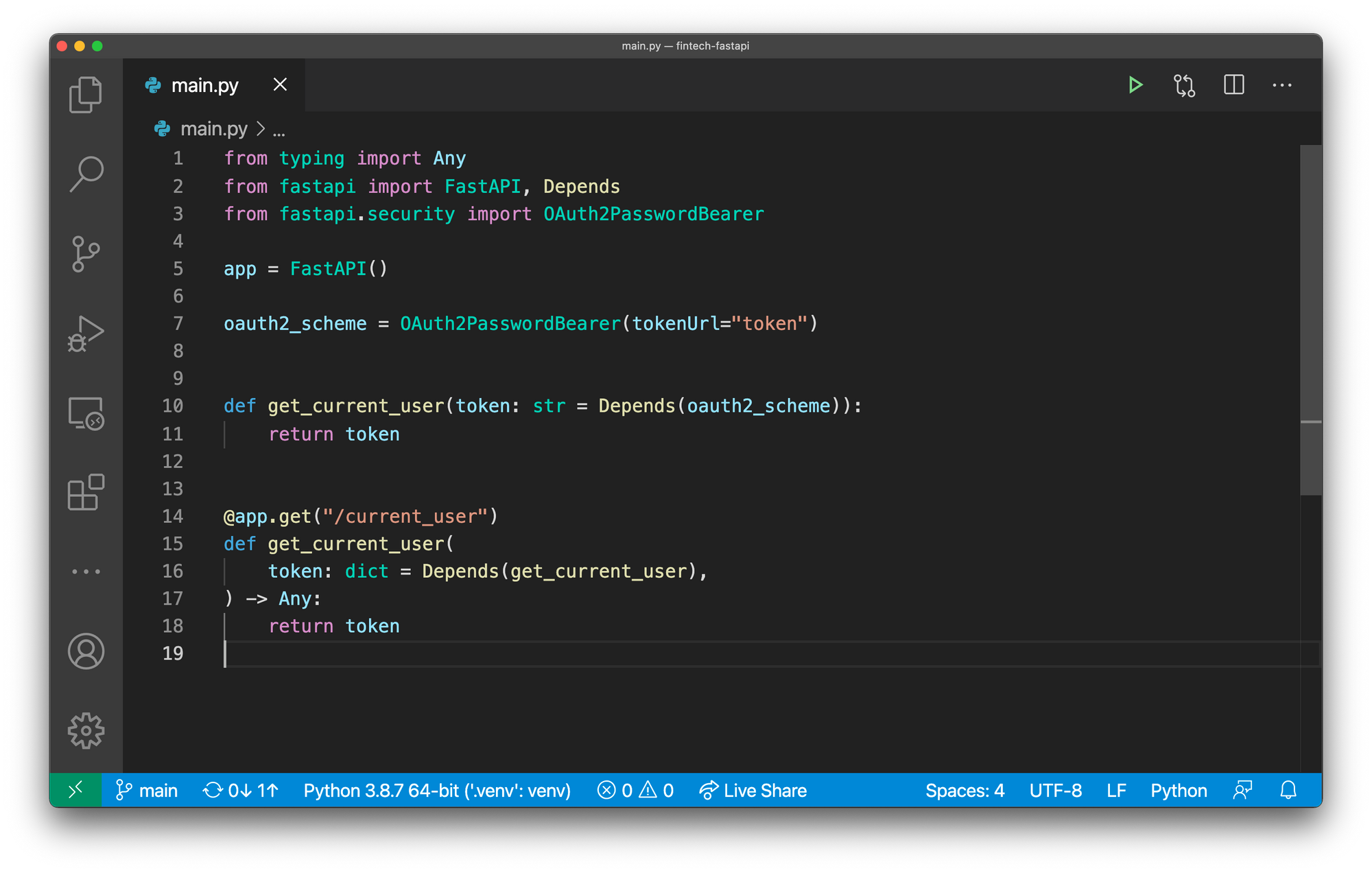
- Testing
- Debugging
This will be just scratching the surface of what FastAPI can do. Be sure to read through the FastAPI User Tutorial and Advanced Tutorial for more info.
Next: Easy FastAPI Setup
Course Schedule
If you want to be notified of when each video comes out you can subscribe to the DeadbearCode YouTube channel where the videos will be posted.
Subscribe
Join the conversation.